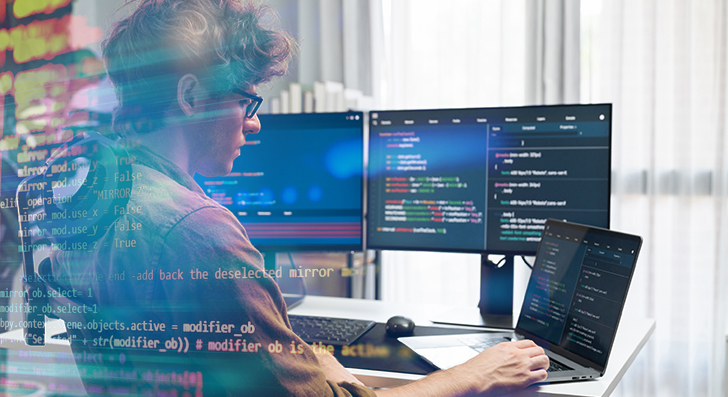
Scalability implies your software can take care of progress—much more people, a lot more information, and more targeted visitors—devoid of breaking. Like a developer, building with scalability in your mind saves time and strain afterwards. Listed here’s a transparent and functional manual to help you start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability isn't really anything you bolt on later—it ought to be part of your respective strategy from the start. Many apps fail every time they expand speedy since the first design and style can’t cope with the extra load. Being a developer, you need to Consider early about how your technique will behave stressed.
Begin by coming up with your architecture to be versatile. Prevent monolithic codebases exactly where almost everything is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into lesser, independent elements. Just about every module or services can scale By itself without affecting The entire process.
Also, give thought to your database from day a single. Will it will need to take care of a million end users or merely 100? Pick the right kind—relational or NoSQL—depending on how your facts will improve. Approach for sharding, indexing, and backups early, Even though you don’t need to have them nevertheless.
A different vital issue is to avoid hardcoding assumptions. Don’t create code that only functions below existing disorders. Think about what would happen if your user foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use style and design patterns that assist scaling, like concept queues or function-driven techniques. These aid your app handle more requests without getting overloaded.
Whenever you Develop with scalability in mind, you are not just planning for achievement—you are lowering potential head aches. A well-prepared system is less complicated to take care of, adapt, and expand. It’s far better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the suitable databases can be a important part of setting up scalable apps. Not all databases are developed exactly the same, and utilizing the Improper one can gradual you down or even bring about failures as your app grows.
Get started by knowledge your info. Can it be hugely structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is a great match. These are sturdy with relationships, transactions, and regularity. They also assist scaling methods like examine replicas, indexing, and partitioning to deal with extra targeted visitors and info.
In the event your info is a lot more flexible—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing big volumes of unstructured or semi-structured facts and can scale horizontally a lot more conveniently.
Also, think about your read through and write designs. Are you presently undertaking many reads with fewer writes? Use caching and browse replicas. Will you be managing a hefty publish load? Check into databases that can manage substantial generate throughput, and even celebration-centered data storage methods like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You may not want Innovative scaling features now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your facts depending on your access patterns. And always check database overall performance as you develop.
In brief, the correct database depends upon your app’s construction, speed requirements, and how you expect it to grow. Choose time to select correctly—it’ll preserve plenty of problems later.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, every single modest delay provides up. Improperly prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s crucial to build economical logic from the beginning.
Begin by writing clean up, basic code. Stay away from repeating logic and take away nearly anything unneeded. Don’t choose the most elaborate Alternative if an easy 1 is effective. Maintain your functions small, targeted, and straightforward to test. Use profiling resources to uncover bottlenecks—spots exactly where your code requires much too extended to operate or employs an excessive amount of memory.
Upcoming, examine your databases queries. These usually gradual issues down much more than the code itself. Make certain Just about every query only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find particular fields. Use indexes to hurry up lookups. And avoid accomplishing too many joins, Specially throughout big tables.
In case you notice the identical facts being requested time and again, use caching. Retail store the outcomes briefly utilizing equipment like Redis or Memcached therefore you don’t have to repeat pricey operations.
Also, batch your databases functions after you can. Rather than updating a row one after the other, update them in teams. This cuts down on overhead and would make your application more successful.
Make sure to exam with large datasets. Code and queries that function fantastic with one hundred data could crash every time they have to take care of one million.
In short, scalable apps are fast apps. Maintain your code restricted, your queries lean, and use caching when necessary. These methods enable your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to deal with a lot more consumers plus more targeted visitors. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two resources assist keep your application rapid, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout several servers. As opposed to 1 server doing all the do the job, the load balancer routes people to diverse servers determined by availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can deliver traffic to the Other folks. Equipment like Nginx, HAProxy, or cloud-primarily based options from AWS and Google Cloud make this straightforward to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers ask for a similar facts once again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could provide it in the cache.
There's two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for speedy accessibility.
two. Customer-side caching (like browser caching or CDN caching) merchants static data files near to the person.
Caching minimizes databases load, improves pace, and makes your app additional effective.
Use caching for things that don’t transform often. And constantly make sure your cache is up to date when facts does alter.
Briefly, load balancing and caching are easy but highly effective resources. Jointly, they assist your app cope with more consumers, stay quickly, and Get better from issues. If you intend to improve, you will need both equally.
Use Cloud and Container Resources
To develop scalable applications, you will need instruments that permit your application grow very easily. That’s the place cloud platforms and containers come in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require here them. You don’t really have to buy components or guess future capacity. When visitors boosts, you may insert extra means with just some clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability instruments. You could center on making your application instead of running infrastructure.
Containers are A different critical Device. A container deals your app and all the things it really should operate—code, libraries, settings—into one device. This can make it effortless to move your application involving environments, out of your laptop to your cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of multiple containers, applications like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your app crashes, it restarts it automatically.
Containers also help it become simple to different portions of your app into services. You may update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to improve with out limits, commence applying these resources early. They help save time, reduce threat, and assist you stay focused on making, not fixing.
Check Anything
If you don’t check your software, you received’t know when things go Improper. Monitoring can help the thing is how your app is undertaking, location issues early, and make far better selections as your application grows. It’s a vital part of creating scalable programs.
Get started by tracking fundamental metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just monitor your servers—watch your application far too. Regulate how much time it's going to take for buyers to load internet pages, how frequently faults happen, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. Such as, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you ought to get notified right away. This assists you repair issues speedy, generally ahead of end users even recognize.
Monitoring is usually handy if you make adjustments. If you deploy a brand new aspect and find out a spike in problems or slowdowns, you are able to roll it again ahead of it triggers real problems.
As your app grows, visitors and information maximize. With no monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal equipment set up, you keep on top of things.
In a nutshell, monitoring will help you keep your application reliable and scalable. It’s not almost recognizing failures—it’s about comprehension your system and making certain it really works effectively, even stressed.
Last Views
Scalability isn’t just for big firms. Even small apps will need a strong Basis. By building very carefully, optimizing sensibly, and using the appropriate applications, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Get started tiny, Assume big, and Construct clever.